Object oriented PHP5: In the land of the blind...

I have read a large number of tutorials on Object Oriented PHP, but for some reason I wasn’t quite getting it.
The principles seem straightforward enough. In layman’s terms—that is to say terms somebody like an English professor with no Comp. Sci. but a lot of XML and procedural PHP experience might understand—Objects are basically groups of related functions and variables.
There is also no problem using one of them. You start a new instance of a class and then feed it information. So here’s an example:
<?php //Prepare class class addition { // This is the method (i.e. function) for this class (you can have more than one). function add($num1, $num2){ $sum = $num1 + $num2; $result = "The sum is " . $sum; return $result; } } //Instantiate a new object $obj_addition = new addition(); // call the add() function with some numbers and assign it to the $sum variable $sum = $obj_addition->add("2", "4"); // Echo the result echo $sum; ?>
This produces
The sum is 6
The problem I had with this seems silly in retrospect. But basically, what I needed to know was “So what? How do I actually use this in a script?”
I can see how that might be useful in a one object script: if I need to know what 2 + 4 is, I understand perfectly how to use this object to discover this. I also understood how to instantiate multiple classes of the same object (though that’s not useful in the above example) so that I could run different sets of variables through the same functions, e.g., with a slightly different class
<?php //Prepare class class addition { // These are the variables required by the class--they need to be provided by the script that instantiates the class. var $num1; var $num2; //This is the function function add(){ $sum = $this->num1 + $this->num2; $result = "The sum is " . $sum; return $result; } } // Instantiate a new object $obj_addition1 = new addition(); // Provide the variables the class needs $obj_addition1 -> num1 = 2; $obj_addition1 -> num2 = 4; // call the add() function with the supplied variables and assign it to $sum1 $sum1 = $obj_addition1->add(); // Instantiate a second object $obj_addition2 = new addition(); // Provide new variables for the new object $obj_addition2 -> num1 = 6; $obj_addition2 -> num2 = 3; // call the add() function with the supplied variables and assign it to $sum2 $sum2 = $obj_addition2->add(); //echo the results echo "<html><body><p>"; echo "\$obj_addition1: " . $sum1 . "<br/>"; echo "\$obj_addition2: " . $sum2; echo "</p></body></html>"; ?>
which produces
$obj_addition1: The sum is 6 $obj_addition2: The sum is 9
But to be honest this isn’t that different from what you could do with a regular function that is just called twice, e.g.
<?php //Build function function add($num1,$num2) { $sum = $num1 + $num2; $result = "The sum is " . $sum; return $result; } //call the function once with some variables $sum1 = add(2,4); //call the function again with a different set of variables $sum2 = add(6,3); echo "<html><body><p>"; echo "\$obj_addition1: " . $sum1 . "<br/>"; echo "\$obj_addition2: " . $sum2; echo "</p></body></html>"; ?>
This is also not what one does mostly in a PHP function, at least not in the ones I make. In my case, I generally need to run a single set of values through multiple functions—open a database, extract some values, process them, return some results, put them back in the database.
So how do you do this? It isn’t very difficult, but in my experience, most tutorials concentrate on the instantiation of one or more instances of the same object rather the processing of values through one or more different objects.
In fact, the problem is this focus on instantiation. The instantiation variable ($variable=new object
) is not the thing to be paying attention to: it is basically the same as an include_once
call for a function saved in a different file. Instead, the real meat of an object oriented script is the variables you use to assign the results of the internal functions (“methods”).
So consider the following, which is basically the same as the above script with an additional object:
<?php //Prepare addition class class addition { // These are the variables required by the class--they need to be provided by the script that instantiates the class. var $num1; var $num2; //This is the function function add(){ $sum = $this->num1 + $this->num2; $result = $sum; return $result; } } //Prepare multiplication class class multiplication { // These are the variables required by the class--they need to be provided by the script that instantiates the class. var $num1; var $num2; //This is the function function multiply() { $product = $this->num1 * $this->num2; $result = $product; return $result; } } //Instantiate new addition object $obj_addition = new addition(); //Instantiate new multiplication object $obj_multiplication = new multiplication(); //Supply some variables to the addition object $obj_addition -> num1 = 2; $obj_addition -> num2 = 4; //call the function $sum = $obj_addition->add(); //manipulate the results $sum2 = $sum - 3; //Supply some variable required by the object $obj_multiplication -> num1 = $sum; $obj_multiplication -> num2 = $sum2; //call the function $product = $obj_multiplication->multiply(); //supply some new variables based on our results $obj_multiplication -> num1 = $sum; $obj_multiplication -> num2 = $product; //Run the class function (method) again with the new values $result= $obj_multiplication->multiply(); //output the results echo "<html> <body> <p>\$sum ($sum) × \$sum ($sum) × \$sum2 ($sum2) = \$sum ($sum) × \$product ($product) = \$result ($result)</p> </body> </html>"; ?>
And this produces:
$sum (6) × $sum (6) × $sum2 (3) = $sum (6) × $product (18) = $result (108)
In the end, Object Oriented PHP is not that hard. But I think for the most part the tutorials concentrate on the bits that are pretty much obvious and ignore the bits that are simple once you understand them but difficult to get your head around if you are used to procedural code. Based on my experience at least, the main thing is not to focus on the instantiation of the class—which is a relatively minor issue. If you treat the instantiation like an include_once()
function, I find that the concepts are not too hard to deal with.
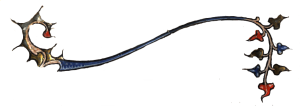
Comment [3]
Paul Richards (Tue May 1, 2007 (21:45:09)) [PermLink]: The power in OO is realized thru inheritance (of parent attributes and behavior), and polymorphism (same Named methods+args) supporting thus generic structure and behavior which is fine tuned at the child class level.
A more extensive example would be required to denote/show it’s power. more later, if you desire. pricha01@hotmail.comdan (Fri May 11, 2007 (17:25:10)) [PermLink]: There are lots of discussions of both these aspects all over the web; but they also tend to suffer from the same problem: showing the easy stuff but not how they are actually used in practice. I’d love some examples if there were some.
Ben Tremblay (Tue Feb 12, 2008 (13:00:15)) [PermLink]: SideBar: apres WildRoseCountry
Well, I was going to kvetch about “no coords on your WikiPedia UserPage?!” but, heh, since you’re into PHP and OOP I’ll let that slide.
I’ll make this short; I don’t want to hijack this thread.
I’ve been trolling for an academic to be the “hero” for my knowledge project. (It’s in stealth mode, but I’ll say this: it might result in something like a Lois Hole Center for Democracy and Community … working title … first time I’ve blurted it publically.)
Would you do me a kindness and peek http://bentrem.sycks.net/gnodal/ for a first impression?
My point is, I’ve been beavering away at IT in the public interest (Google “participatory deliberation” and my site might be #1) since 1975 … and, w/respect to such as WikiPedia, we ain’t seen nuthin’ yet.regards
—bentrem
http://bentrem.sycks.net